Hookdeck TypeScript SDK in Public Beta
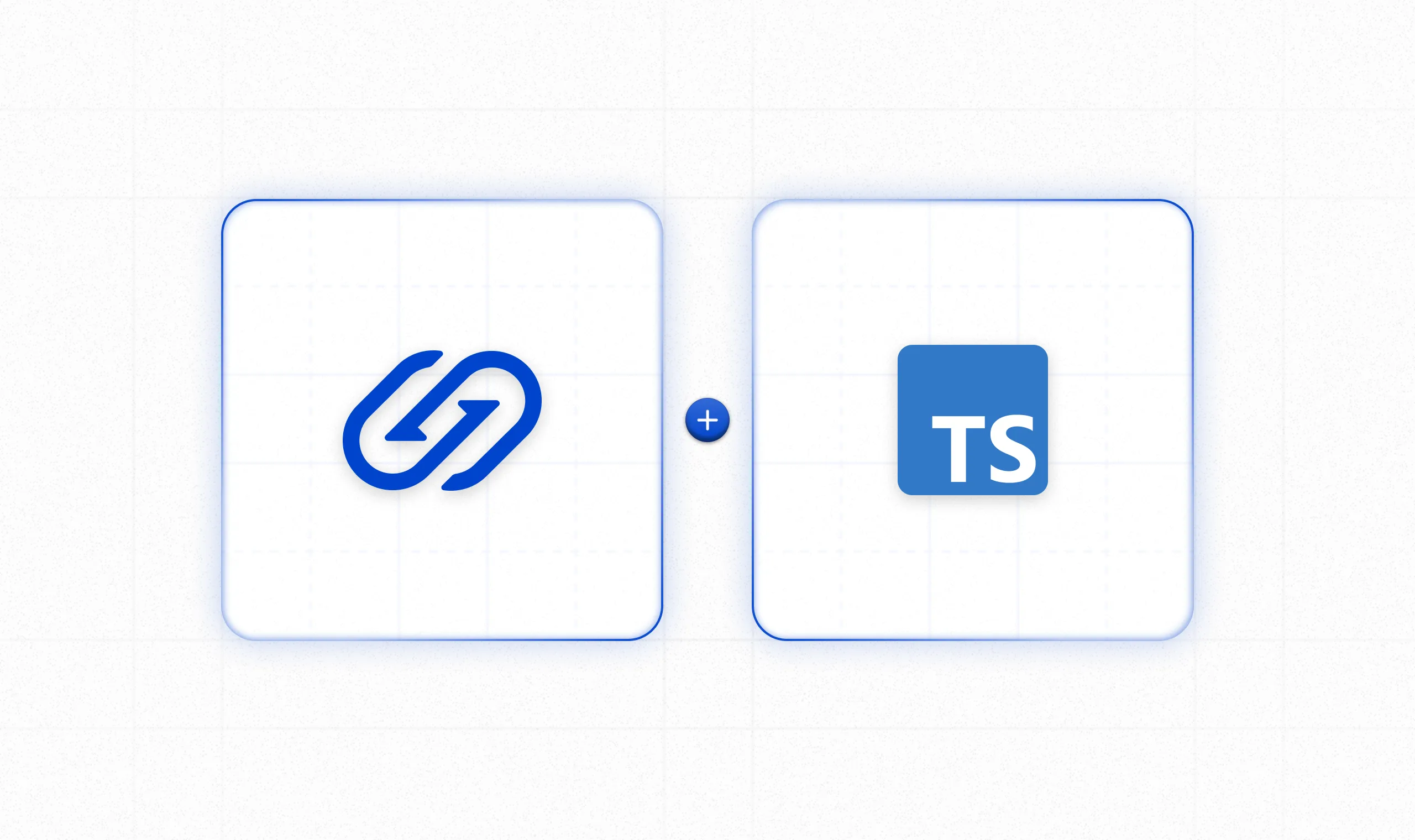
The Hookdeck TypeScript SDK is now in public beta. The SDK enables you to build for use cases that require dynamic orchestration of Hookdeck connections (sources, authentication, transformations, filters, routing, queueing, destinations, rate-limiting, etc.), such as adding outbound webhook support to your event-driven applications. The release of the TypeScript SDK alongside the recently released Go SDK improves the Hookdeck developer experience further by providing a more idiomatic way to interact with the Hookdeck API.
The Hookdeck TypeScript SDK is intended for non-browser runtimes such as Deno or Node.js.
Both Hookdeck SDKs are built with Fern via an automation triggered by changes to our OpenAPI specification.
Using the Hookdeck TypeScript SDK
You can get started with the Hookdeck TypeScript SDK by installing it from JSR or NPM:
deno add @hookdeck/sdk
npx jsr add @hookdeck/sdk
yarn dlx jsr add @hookdeck/sdk
npm install @hookdeck/sdk
yarn add @hookdeck/sdk
Once installed, you need a Hookdeck Project API Key, and then you can initialize a new instance of the SDK as follows:
import { HookdeckClient } from '@hookdeck/sdk';
const hookdeck = new HookdeckClient({
token: 'HOOKDECK_API_KEY'
});
With the SDK initialized, you can now interact with the Hookdeck API.
For example, in the outbound webhook scenario, upon registration of a customer URL for an outbound webhook, you can create a connection:
const createWebhookCallback = async ({customerId, eventType, callbackUrl}) => {
const connection = await hookdeck.connection.create({
name: "outbound-example",
source: {
name: "trigger"
},
destination: {
name: `${customerId}-${eventType}-outbound`",
url: callbackUrl
}
});
await storeInDb({
customerId,
eventType,
sourceUrl: connection.source.url
});
}
When an event occurs within a system, and you want to trigger a webhook, you can use the SDK to retrieve the Hookdeck URL to send the event:
const eventTriggered = async ({ customerId, eventType, eventData }) => {
const { sourceUrl } = getFromDb(customerId, eventType);
await fetch(sourceUrl, {
method: 'POST',
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify(eventData),
});
};
By doing this, you use Hookdeck as your infrastructure for queuing, guaranteed at-least-once delivery, rate limiting, and more.
Beta Feedback
If you find a problem with the SDK or have any functional requests, please open an issue on the SDK's GitHub repository or join the Hookdeck community Slack and let us know.